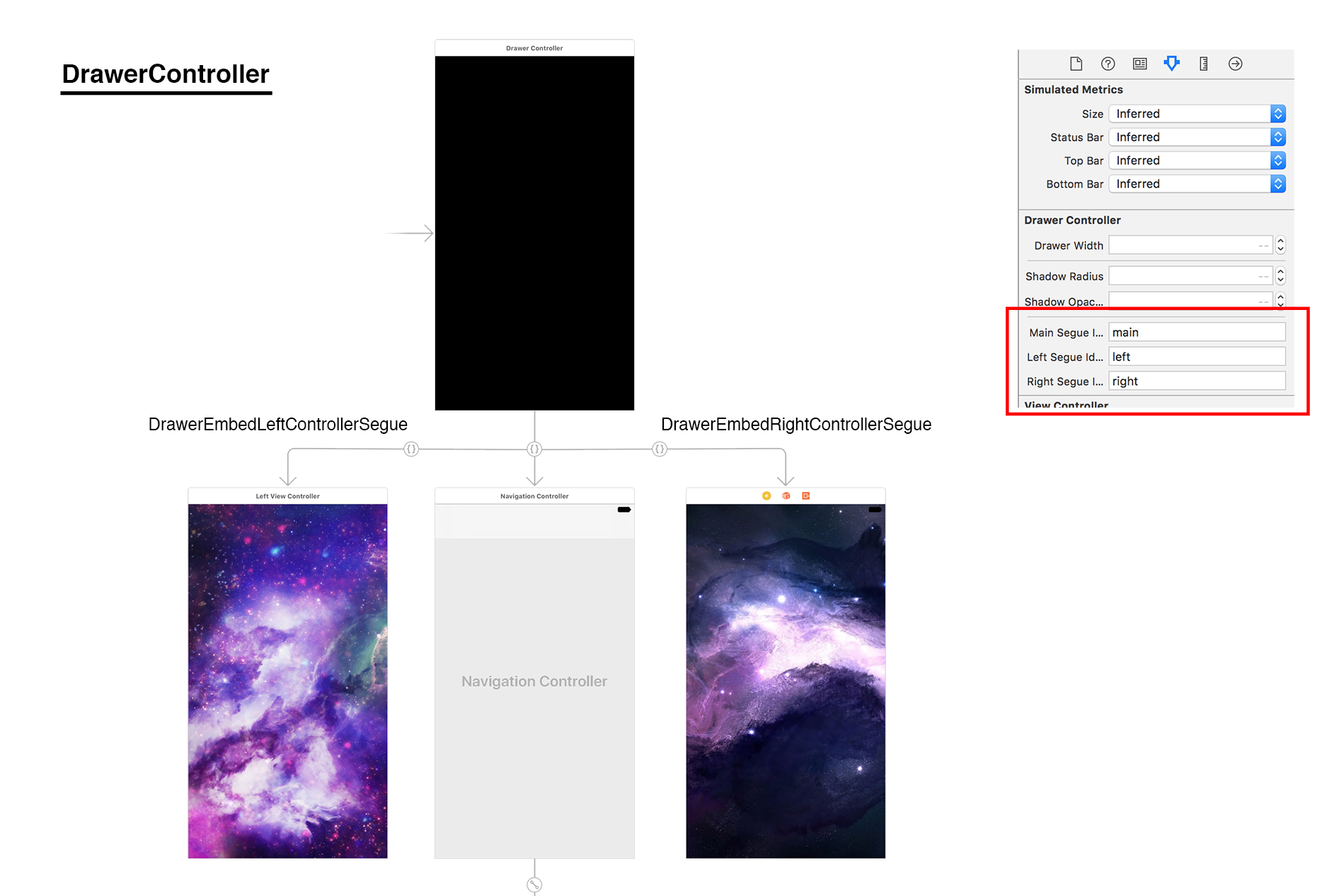
A drawer view controller is an iOS class that makes it easy to create a collection of controllers. It wraps all the animations and other UIViewController-like features in one place. The result is much less code, which results in faster UI development time for your app.
The “kydrawercontroller” is a drawer view controller that easy to use. It can be used with UIViewController and Xcode 7.
A simple to use drawer view controller!
Installation
CocoaPods (projects for iOS 8 and above)
On CocoaPods, you may find KWDrawerController. To your Podfile, add the following:
# Swift 3 pod ‘KWDrawerController’, ‘> 3.7’ # Swift 4 pod ‘KWDrawerController’, ‘> 4.2’ pod ‘KWDrawerController/RxSwift’
Manually
Drag the DrawerController folder into your existing project and put it in.
Usage
Code
@UIApplication import UIKit import KWDrawerController func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) main class AppDelegate: UIResponder, UIApplicationDelegate var window: UIWindow? -> let mainViewController = MainViewController() let leftViewController = LeftViewController() let rightViewController = RightViewController() let drawerController = DrawerController() drawerController let mainViewController = MainViewController() drawerController.setViewController(mainViewController,.none) drawerController.setViewController(leftViewController,.left) viewController is set (rightViewController, .right) Window = UIWindow(frame: UIScreen.mainScreen().bounds) window customizing? . drawerController window = rootViewController? . return true if makeKeyAndVisible() is true
Storyboard
-
Set the Initial ViewController’s Custom Class to DrawerController.
-
Connect DrawerController’s DrawerEmbedLeftControllerSegue and/or DrawerEmbedRightControllerSegue to your left/right controllers.
-
Connect the DrawerEmbedMainControllerSegue from DrawerController to your main controller using the DrawerEmbedMainControllerSegue.
-
Set the segue IDs for both the inspector and the segues themselves in DrawerController.
Close / Open
/ openSide self.drawerController? (.left) self.drawerController?.openSide(.right) / closeSide self.drawerController? ()
Delegate
drawer function (optional) DidAnimation happen? ( drawerController: DrawerController, side: DrawerSide, percentage: Float ) drawer function (optional) DidAnimationBegin ( drawerController: DrawerController, side: DrawerSide ) drawer function (optional) WillFinishAnimation is a placeholder for WillFinishAnimation, which ( drawerController: DrawerController, side: DrawerSide ) drawer function (optional) WillAnimationBeCancelled ( drawerController: DrawerController, side: DrawerSide ) drawer function (optional) DidFinishAnimationFinishAnimationFinishAnimationFinish ( drawerController: DrawerController, side: DrawerSide ) drawer function (optional) DidAnimationCancel ( drawerController: DrawerController, side: DrawerSide )
Customizing
Transition
DrawerTransition is a module that determines the Drawer’s rendering direction. It’s done by inheriting from DrawerTransition.
- DrawerScaleTransition
- DrawerParallaxTransition
- DrawerFloatTransition
- Transition from Overflow should also utilize DrawerFloatTransition while utilizing the Transition.
Overflow Transition
When transitioning beyond the drawer’s open range, an overflow transition should be applied.
- DrawerSlideTransition
- DrawerScaleTransition
- When using DrawerSlideTransition, DrawerParallaxTransition, DrawerFoldTransition, and DrawerSwingTransition, this is normal.
- DrawerParallaxTransition
- DrawerFloatTransition
- Transition should additionally utilize DrawerFloatTransition when employing the Overflow Transition.
- DrawerFoldTransition
- DrawerSwingTransition
- DrawerZoomTransition
Animator
The Animator module regulates the pace at which a drawer moves. DrawerAnimator or DrawerTickAnimator are used to implement it.
- DrawerLinearAnimator
- DrawerCurveEaseAnimator
- DrawerSpringAnimator
- DrawerCubicEaseAnimator
- DrawerQuadEaseAnimator
- DrawerQuartEaseAnimator
- DrawerQuintEaseAnimator
- DrawerCircEaseAnimator
- DrawerExpoEaseAnimator
- DrawerSineEaseAnimator
- DrawerElasticEaseAnimator
- DrawerBackEaseAnimator
- DrawerBounceEaseAnimator
Options
is a public variable Bool public var is TapToClose: Bool public var is a gesture. Bool public var is animated Overflow Bool public var is animated bool public var is bool public var is bool public var is bool public Bool public var is FadeScreen Bool public var is a bool bool bool bool bool bool Enable: Bool
Changelog
- 1.0 is the first release.
- 1.1 Bug fixes and animations have been included.
- Refactoring 2.0
- 2.1 Bug fix and animation update.
- 2.2 Improved animation and fixed a few problems.
- 3.0 Developed in Swift 3.0
- 3.1 DrawerController’s Access Control concerns have been resolved.
- 3.2 On Transition, fix the Access Control problems.
- 3.3 On the initializer, fix the Access Control concerns.
- 3.4 Discard the debug log.
- 3.5 Fixed an issue in which “Absolute Controller” did not apply touch ignores.
- 3.6 Fixed a problem that occurred while the drawer was open and the layout was changing.
- 3.6.1 Fixed a layout problem that occurred when the device was rotated.
- 3.7 Issues with properties not updating were fixed.
- Swift 4 is supported in version 4.0.
- 4.1 Add a new flag that permits auto-direction switching.
- 4.1.1 RxSwift support (If you want).
- 4.1.2
- Fix difficulties with child view controllers’ auto layout.
- Names should be replaced.
- Use the getViewController function to get a view controller.
- Reduce the size of the cloning process.
- 4.1.3
- Crash on load has been fixed. (#12)
- 4.1.4
- To delegate, add state methods. (#16)
- (#18) Resolve access control concerns.
- DrawerFloatTransition problem has been fixed (#20).
- The lifecycles of child controllers are wrongly managed by DrawerController. #22 (#21)
- 4.1.5
- Improvements to the code and performance, as well as bug fixes. (@rivera-ernesto) #24
- 4.1.6
- Fix the transition issues.
- Bugs with gestures that don’t function should be fixed.
- Correct iPad drawer arrangement (#28 @rivera-ernesto)
- 4.2
Requirements are a set of rules that must be followed.
Permission
The MIT license applies to KWDrawerController. For further information, see the LICENSE file.
GitHub
https://github.com/Kawoou/KWDrawerController
The “ios slide view up from bottom github” is a drawer view controller that easy to use. The developer can easily add this type of drawer into their project.
Related Tags
- mmdrawercontroller
- draggable view controller swift github
- slide view controller swift
- ios side menu example
- material design navigation drawer github